I Need Python Code For Analyze, Explain, And Present The CHCxcN8
A machine learning project entails well-known steps such as defining the problem, preparing data, and evaluating algorithms. The describe() function in Python computes basic statistical values for a dataset. The Train/Test method is used to measure the accuracy of a model. Python is a popular language for data analytics, and this article discusses its use case with a demo. It also explains the main steps of a classification use case using data science and Python. Additionally, there are over 180 solved data science and machine learning projects using Python, along with frameworks and web apps to interpret and explain ML models. This tutorial introduces neural networks and artificial intelligence in Python.
To analyze, explain, and present the results of a dataset in AI and machine learning using Python, you can follow the steps below:
Step 1: Data Preparation and Analysis
import pandas as pd
# Load the dataset
data = pd.read_csv('your_dataset.csv')
# Analyze the data
print(data.head()) # View the first few rows of the dataset
print(data.describe()) # Get statistical information about the dataset
Step 2: Machine Learning Model
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Prepare the data for training
X = data.drop('target_column', axis=1) # Features
y = data['target_column'] # Target variable
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Initialize a machine learning model
model = RandomForestClassifier()
# Train the model
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, predictions)
print("Model Accuracy: ", accuracy)
Step 3: Presentation and Explanation
import matplotlib.pyplot as plt
# Visualize the results
# Example: Plot feature importances
feature_importances = pd.Series(model.feature_importances_, index=X.columns)
feature_importances.nlargest(10).plot(kind='barh')
plt.show()
In this code, you should replace 'your_dataset.csv' with the actual filename of your dataset and 'target_column' with the name of the column you want to predict. The RandomForestClassifier is just an example; you can use other models depending on your specific use case.
Feel free to ask for additional help or clarification on any of these steps!
Sources
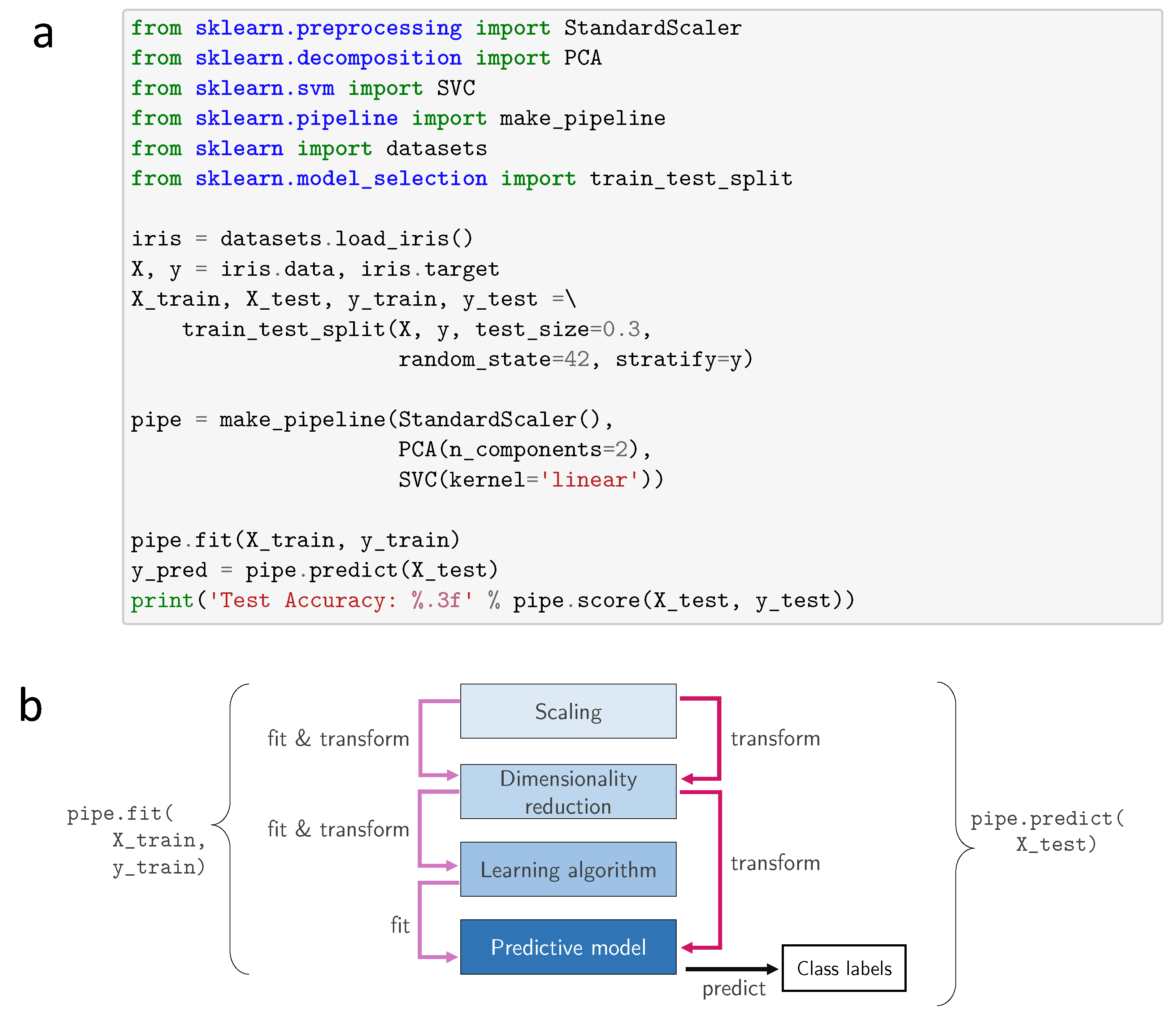
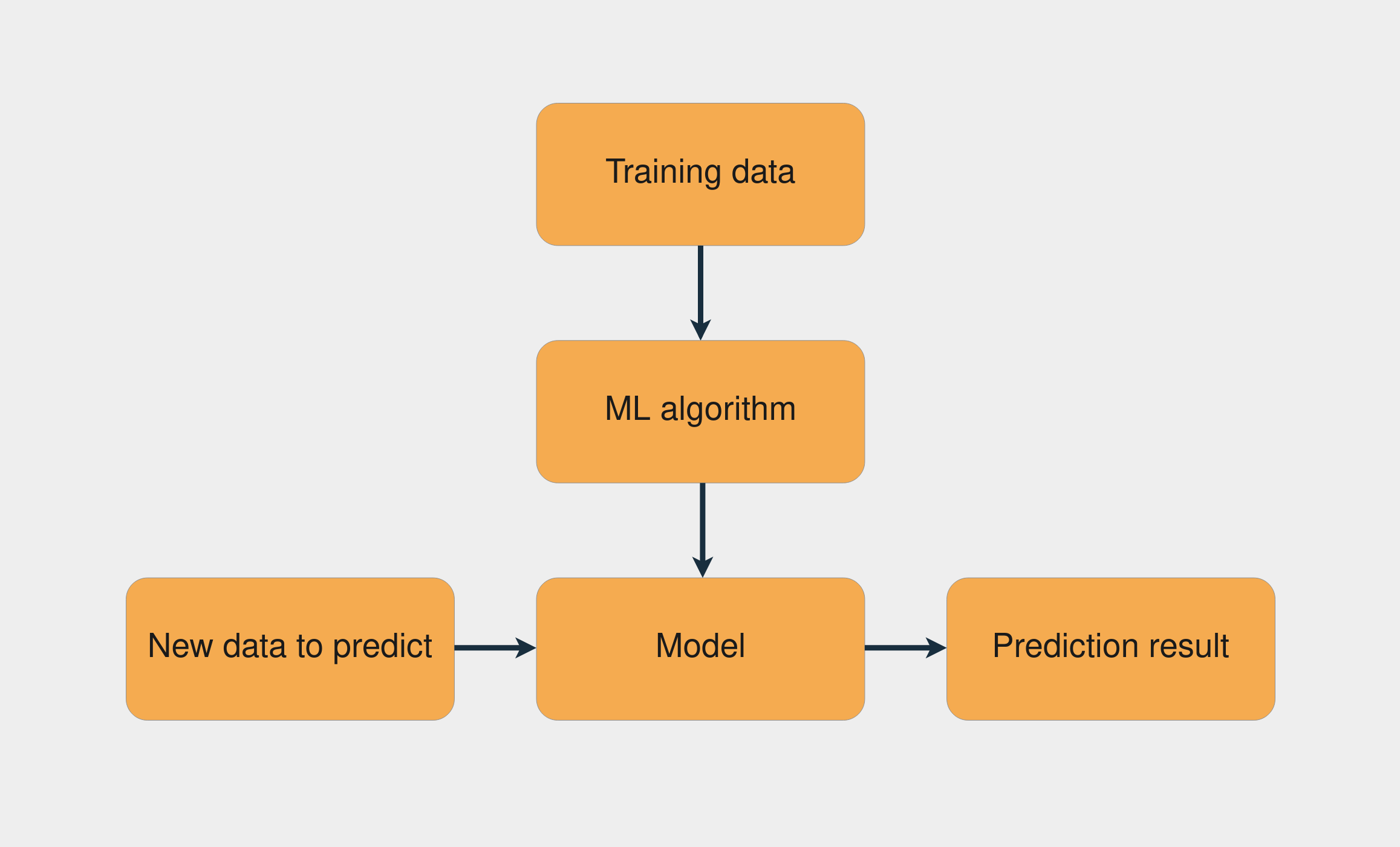
Related Questions
Work fast from anywhere
Stay up to date and move work forward with BrutusAI on macOS/iOS/web & android. Download the app today.