Find The Prime Numbers In Python Profram 6JQr87B
To check if a number is prime in Python, a basic approach is used where all numbers from 2 to the square root of the given number plus one are searched for factors. Prime numbers are a subset of natural numbers with only 1 and the number itself as factors. The Python library SymPy has functions specifically for generating prime numbers, such as isprime(n). To print a prime number in Python, the program must define a function that takes a number as an argument and returns only prime numbers within a given interval. When checking if a number is prime, it is important to note that the number must be greater than 1 and is divided by all numbers between 2 and the number itself minus one. This can be achieved using a for or while loop.
To generate prime numbers in Python, you can take one of several approaches. Here's the general idea behind identifying prime numbers and a simple program to do so:
def is_prime(num):
if num > 1:
for i in range(2, int(num**0.5) + 1):
if num % i == 0:
return False
return True
# Getting prime numbers in a range
lower_bound = 10
upper_bound = 50
print("Prime numbers between", lower_bound, "and", upper_bound, "are:")
for n in range(lower_bound, upper_bound + 1):
if is_prime(n):
print(n)
In this example, the is_prime
function checks if a number is prime. Then, the program prints prime numbers within a specific range. This method involves checking divisibility up to the square root of the number, making it more efficient than checking up to the number itself.
If you need any further assistance, don't hesitate to ask!
Sources
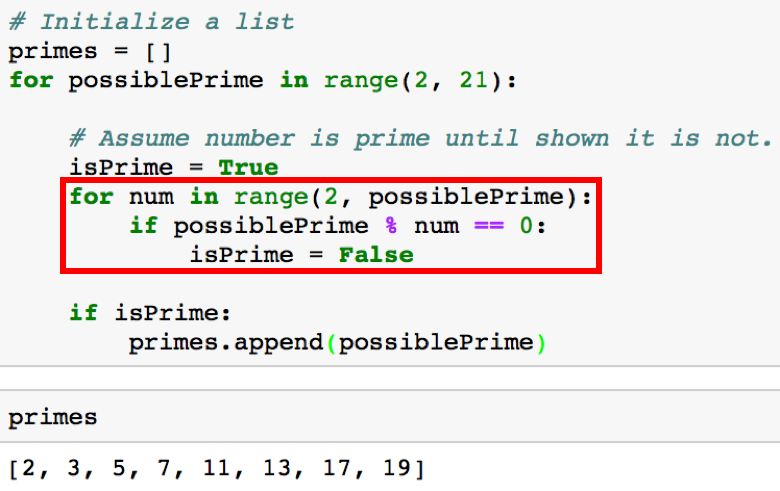
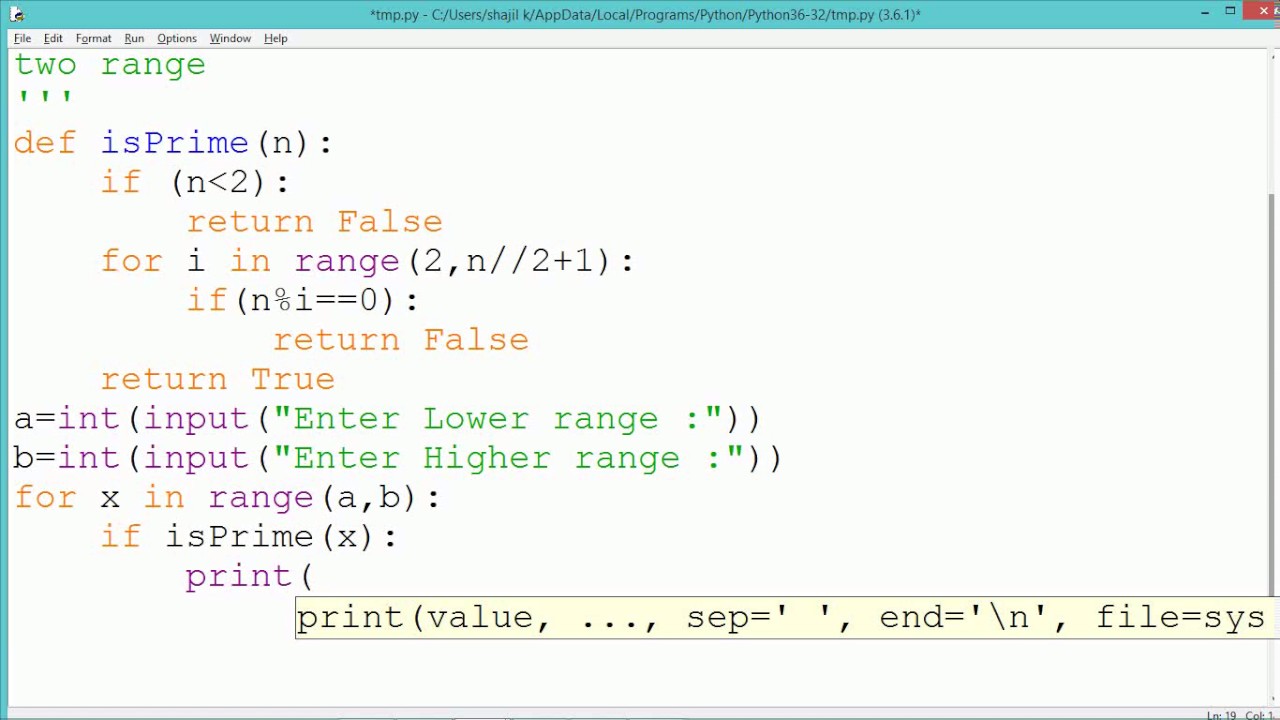
Related Questions
Work fast from anywhere
Stay up to date and move work forward with BrutusAI on macOS/iOS/web & android. Download the app today.